This program computes frequency of characters in a string i.e. which character is present how many times in a string. For example in the string "code" each of the character 'c', 'o', 'd', and 'e' has occurred one time. Only lower case alphabets are considered, other characters (uppercase and special characters) are ignored. You can easily modify this program to handle uppercase and special symbols.
C programming code
Explanation of "count[string[c]-'a']++", suppose input string begins with 'a' so c is 0 initially and string[0] = 'a' and string[0]-'a' = 0 and we increment count[0] i.e. a has occurred one time and repeat this till complete string is scanned.
Output of program:
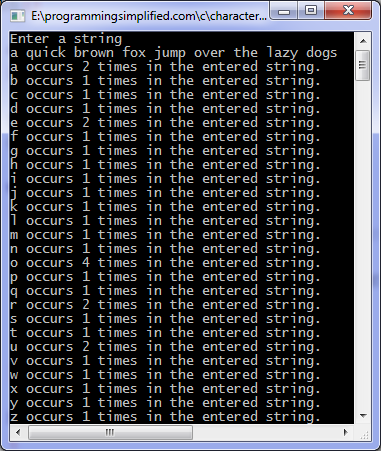
Did you notice that string in the output of program contains every alphabet at least once.
Calculating frequency using function
We will make a function which computes frequency of characters in input string and print in a table (see output image below).
Output of progran:
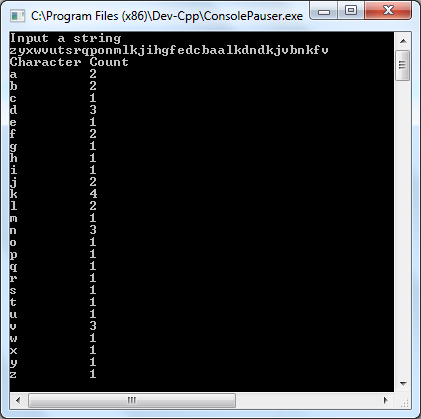
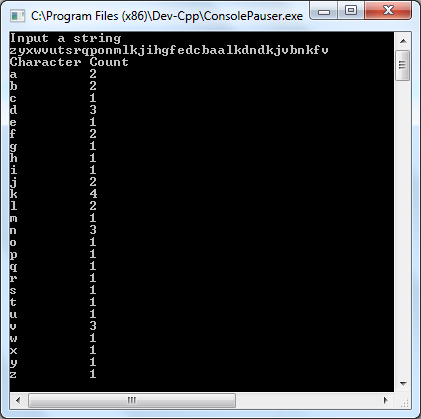
We do not pass no of elements of array count[] to function find_frequency as they will always be 26. But you can pass if you wish to do so.
0 comments:
Post a Comment