C program to sort a string in alphabetic order: For example if user will enter a string "programming" then output will be "aggimmnoprr" or output string will contain characters in alphabetical order. In our program we assume input string contains only lower case alphabets. First we count and store how many times characters 'a' to 'z' appear in input string and then create another string which contains characters 'a' to 'z' as many times as they appear in the input string.
C programming code
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
char ch, input[100], output[100];
int no[26] = {0}, n, c, t, x;
printf("Enter some text\n");
scanf("%s", input);
n = strlen(input);
/** Store how many times characters (a to z)
appears in input string in array */
for (c = 0; c < n; c++)
{
ch = input[c] - 'a';
no[ch]++;
}
t = 0;
/** Insert characters a to z in output string
that many number of times as they appear
in input string */
for (ch = 'a'; ch <= 'z'; ch++)
{
x = ch - 'a';
for (c = 0; c < no[x]; c++)
{
output[t] = ch;
t++;
}
}
output[t] = '\0';
printf("%s\n", output);
return 0;
}
Output of program:
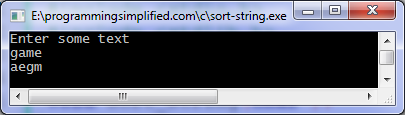
C program using pointers
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void sort_string(char*);
int main()
{
char string[100];
printf("Enter some text\n");
gets(string);
sort_string(string);
printf("%s\n", string);
return 0;
}
void sort_string(char *s)
{
int c, d = 0, length;
char *pointer, *result, ch;
length = strlen(s);
result = (char*)malloc(length+1);
pointer = s;
for ( ch = 'a' ; ch <= 'z' ; ch++ )
{
for ( c = 0 ; c < length ; c++ )
{
if ( *pointer == ch )
{
*(result+d) = *pointer;
d++;
}
pointer++;
}
pointer = s;
}
*(result+d) = '\0';
strcpy(s, result);
free(result);
}
0 comments:
Post a Comment